Building Hitflow.xyz
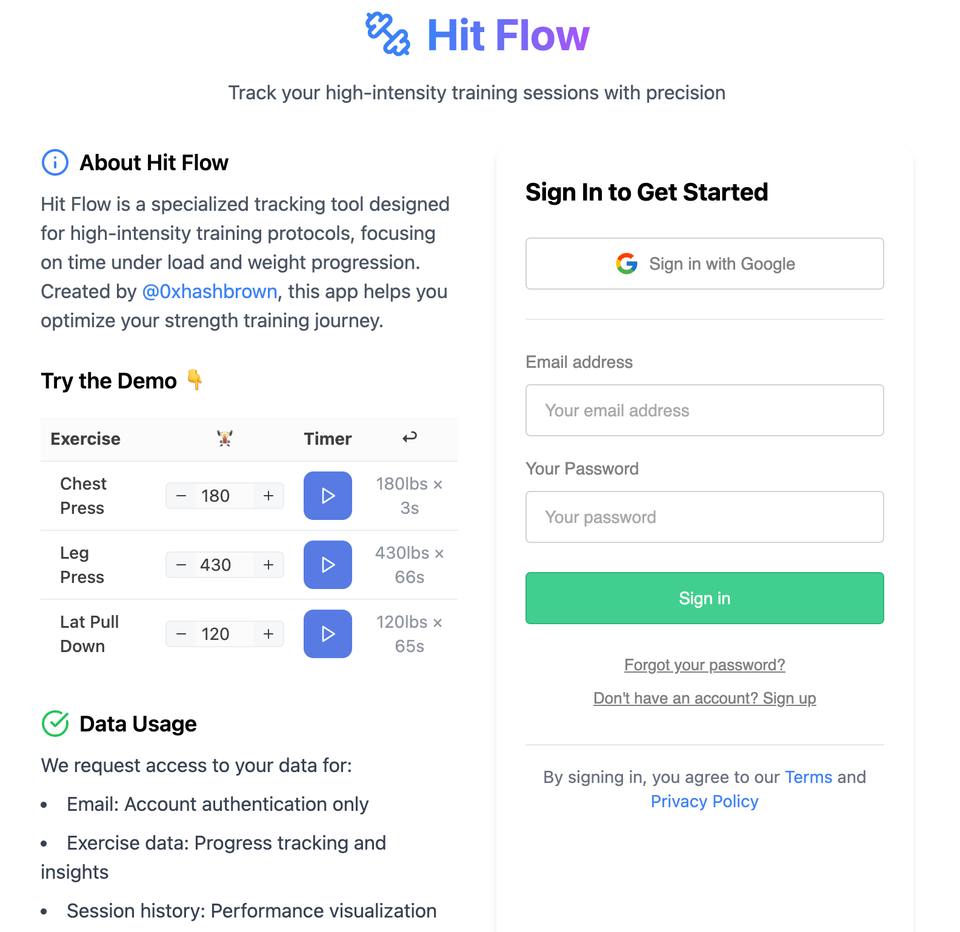
Building a High-Intensity Training Tracker: From AI Prototype to Production App
As a developer with 15+ years of experience, I recently undertook an exciting project to build a High-Intensity Training (HIT) tracker application. This post details my journey from initial concept to a fully functional TypeScript application, leveraging modern tools and AI assistance along the way.
Project Overview
The HIT Tracker is a web application designed to help users track their high-intensity training sessions, focusing on:
- Time under load tracking
- Weight progression
- Visual progress monitoring
- Audio feedback for timing
Tech Stack
The application was built using a modern frontend stack:
- React + TypeScript: For type-safe component development
- Vite: As the build tool and development server
- Tailwind CSS: For rapid, utility-first styling
- Recharts: For beautiful, responsive data visualization
- Lucide Icons: For consistent, scalable iconography
- Local Storage: For client-side data persistence
Development Journey
1. Initial Prototyping with AI
I started by using srcbook.com for initial prototyping. This allowed me to:
- Rapidly iterate on the UI design
- Experiment with different component structures
- Get quick feedback on architectural decisions
The AI assistance helped me:
- Generate boilerplate code
- Suggest component organization
- Identify potential edge cases
- Implement best practices
2. Development Environment
I used Cursor as my primary IDE, which provided:
- Integrated AI assistance
- Smart code completion
- Real-time error detection
- Efficient refactoring tools
3. Core Features Implementation
Timer System
One of the most critical features was the sophisticated timer system, which includes:
- Precise time tracking
- Audio feedback for countdown
- Visual progress indicators
- Customizable settings
// Example of the timer implementation
const [timerState, setTimerState] = useState<TimerState>({
isRunning: false,
time: 0,
exerciseName: null
});
Data Visualization
I implemented comprehensive progress tracking using Recharts:
- Multiple data series
- Time-based progression
- Weight tracking
- Interactive tooltips
State Management
The application uses a combination of:
- React hooks for local state
- localStorage for persistence
- Custom hooks for shared functionality
4. User Experience Considerations
I focused heavily on UX details:
- Clean, intuitive interface
- Responsive design
- Audio feedback
- Helpful tooltips and guidance
- Progressive disclosure of features
5. Performance Optimization
Key optimizations included:
- Efficient re-rendering strategies
- Memoization of expensive calculations
- Lazy loading of non-critical components
- Audio preloading for timing feedback
Challenges and Solutions
1. Timer Precision
Challenge: Maintaining accurate timing across browser tabs
Solution: Implemented a delta-time calculation system
2. Data Persistence
Challenge: Reliable local storage with type safety
Solution: Created typed wrapper functions for localStorage operations
3. Audio Feedback
Challenge: Browser autoplay restrictions
Solution: Implemented user-triggered sound initialization
Lessons Learned
-
AI as a Collaborator
- AI tools are most effective when used to augment human expertise
- Clear requirements and oversight are crucial
- AI can significantly speed up boilerplate tasks
-
TypeScript Benefits
- Strong typing caught numerous potential issues early
- Improved code maintainability
- Better developer experience with autocomplete
-
Modern Web Standards
- Progressive Web App capabilities
- Local storage reliability
- Browser API considerations
Future Improvements
-
Data Sync
- Cloud backup implementation
- Multi-device synchronization
- Offline-first architecture
-
Analytics
- Training pattern analysis
- Progress predictions
- Performance insights
-
Social Features
- Sharing capabilities
- Community challenges
- Progress comparisons
Conclusion
Building the HIT Tracker demonstrated how modern development tools and AI assistance can accelerate the development process without compromising on quality. The combination of experienced development practices with cutting-edge tools created a robust, user-friendly application that serves its purpose effectively.
The project went from concept to production in a week, showcasing the power of:
- Modern development tools
- AI assistance
- Type-safe programming
- Rapid prototyping
- Iterative development
For developers looking to undertake similar projects, I recommend:
- Start with clear requirements
- Leverage AI tools wisely
- Focus on core functionality first
- Maintain type safety
- Prioritize user experience
- Test continuously
The source code is available on GitHub, and I welcome contributions and feedback from the community.
This post was written by a developer with 15+ years of experience in web development, focusing on modern JavaScript/TypeScript applications and UI/UX design.